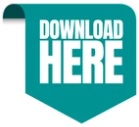
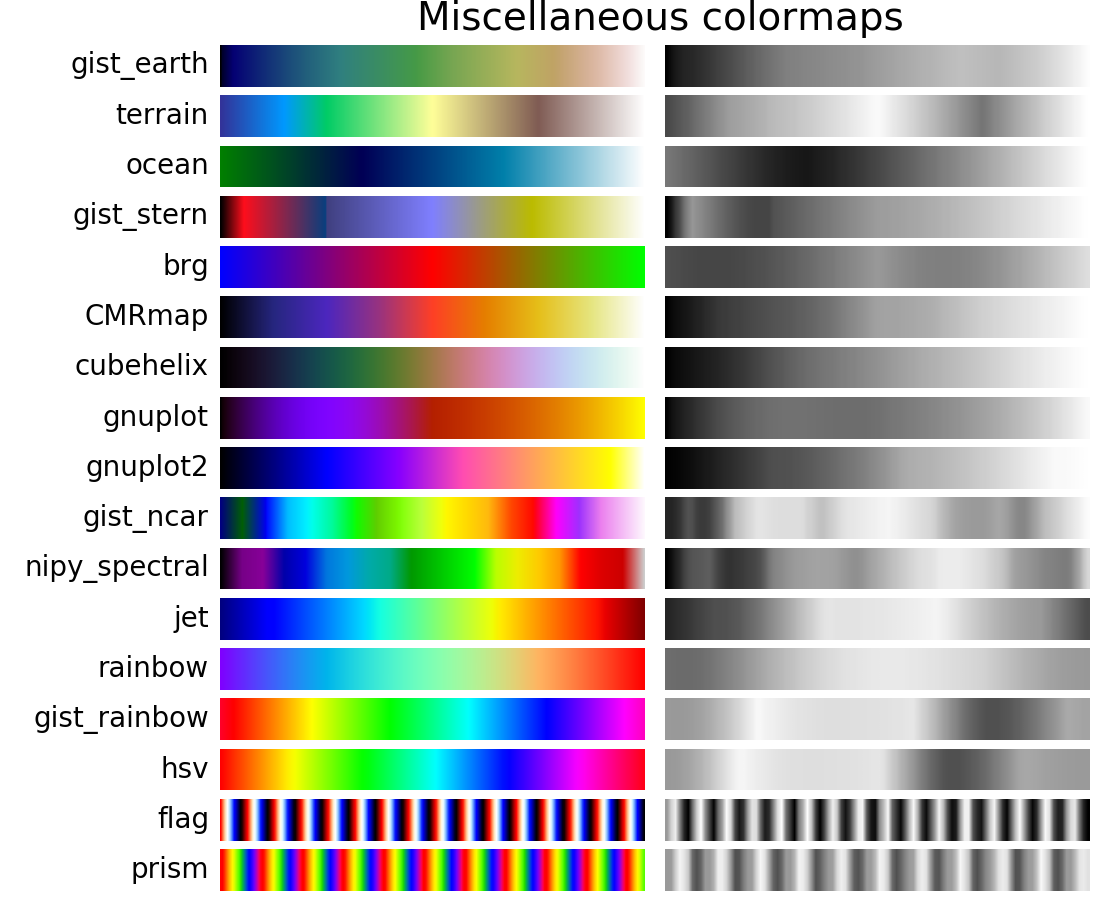
#Python image convert to rgb full#
If you scale your greyscale image to the full range after reading, using this line: grey = ((grey.astype(float) - grey.min())*255/(grey.max() - grey.min())).astype(np.uint8) # Take entries from RGB LUT according to greyscale values in image Result = np.zeros((*grey.shape,3), dtype=np.uint8) # Make output image, same height and width as grey image, but 3-channel RGB Lut = np.loadtxt('cmap.csv', dtype=np.uint8, delimiter=',') Grey = np.array(Image.open('TdoGc.png').convert('L')) # Load image as greyscale and make into Numpy array Looking up can be done by loading the CSV file containing our colourmap, and taking the corresponding elements from the colormap as indexed by your greyscale values in the range 0.255: #!/usr/bin/env python3
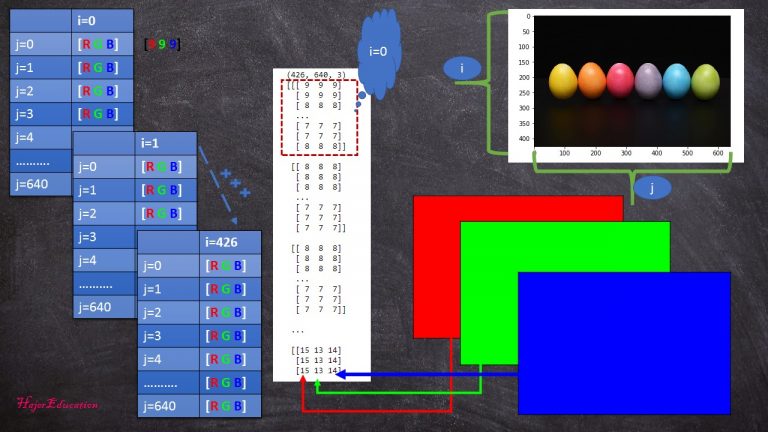
If we now want to scale that from the range 17.317 into the range 0.255, we can use: rescaled = ((grey.astype(float) - grey.min())*255/(grey.max() - grey.min())).astype(np.uint8) Instead then, I will create a dummy 32x32 image with a range of 17.317 like this: grey = np.random.randint(17,318, (32,32)) Also, you have not said how you plan to read a TIFF on a system that doesn't have OpenCV or matplotlib, so I don't know whether you have PIL/Pillow or plan to use tifffile. Regarding scaling, you have posted an 8-bit PNG image with a range of 0.117 rather than a 16-bit TIFF image with a range of 17.317, so that is not most helpful. Presumably you can copy that file to your production machine and you can choose any one of Matplotlibs colormaps. Anywhere we see 1 in the greyscale it maps to rgb(68,2,85). That means anywhere we see 0 in the greyscale image, we actually colour the pixel rgb(68,1,86). If we look at that file "cmap.csv", it has 256 lines and starts like this: 68,1,84 # Each line corresponds to an RGB colour for a greyscale level # Make a Numpy array of the 256 RGB values # Get 256 entries from "viridis" or any other Matplotlib colormap We'll take the viridis colormap: #!/usr/bin/env python3 I understand you can't use matplotlib in production, but you can grab a colormap or two from there on some other machine and copy it to your production machine. We can lookup any greyscale value and find the colour we want to show it as. So, to create a colormap, we need a list of 256 RGB values in which

So, rather than: r_np = np.array(cv2.imread(red, 0))Ĭonsider: r_np = cv2.imread(filename, cv2.IMREAD_GRAYSCALE)Īssert r_np is not None, "ERROR: Error reading red image"Ĭonvert grayscale 2D numpy array to RGB image

#Python image convert to rgb for free#
Secondly, you are using ugly, manifest constants instead of the nice, legible, maintainable ones that OpenCV provides for free and also you are needlessly wrapping the Numpy array you get from cv2.imread() into a Numpy array.įinally, you are not checking for errors. Combine 3 grayscale images to 1 natural color image in Pythonįirstly, you need to import modules: import numpy as np This makes the values not only float32 but also between 0 and 1. Rgb_image = cv2.cvtColor(img,cv2.COLOR_GRAY2RGB) In img = X.astype('float32') #cv2 needs float32 or uint8 for handling images Plt.imshow(gray, cmap=plt.get_cmap('gray'), vmin=0, vmax=1)Ĭonverting a grayscale image to rgb damages imageĬorrect me if I am wrong but I suspect the values in X are in the range 0,255. If an alpha (transparency) channel is present in the input image and should be preserved, use mode LA: img = Image.open('image.png').convert('LA') Img = Image.open('image.png').convert('L') How about doing it with Pillow: from PIL import Image How can I convert an RGB image into grayscale in Python? The hard way (method you were trying to implement): backtorgb = cv2.cvtColor(gray,cv2.COLOR_GRAY2RGB) You could draw in the original 'frame' itself instead of using gray image. How does one convert a grayscale image to RGB in OpenCV (Python)?
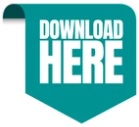